OOPS stands for Object-Oriented Programming (OOP), a programming paradigm that uses “objects” to design and structure programs. In OOP, objects are instances of classes, which are templates for creating objects.
In this article , we will explore core OOP concepts using easy to understand code examples.
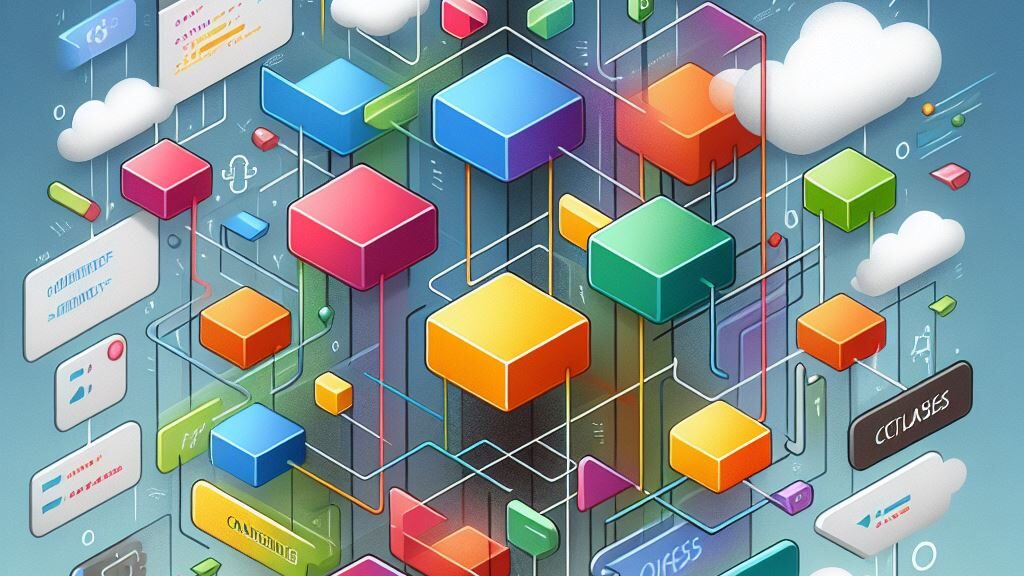
1. Classes and Objects
In object-oriented programming (OOP), classes and objects are fundamental concepts. Here’s a breakdown:
Classes:
- Blueprints: Classes are like blueprints or templates for creating objects. They define the attributes (data) and methods (functions) that objects of that class will have.
- Encapsulation: Classes encapsulate data (attributes) and behavior (methods) into a single unit.
- Reusability: Once a class is defined, you can create multiple objects (instances) of that class.
- Inheritance: Classes can inherit attributes and methods from other classes, allowing for hierarchical organization and code reuse.
- Abstraction: Classes abstract real-world entities by defining their essential properties and behaviors.
Objects:
- Instances of Classes: Objects are instances of classes. When you create an object, you’re creating a specific instance of that class with its own set of data.
- Attributes: Objects have attributes, which are the data associated with them. These attributes are defined by the class.
- Methods: Objects can perform actions through methods, which are functions defined within the class.
- Identity: Each object has a unique identity, distinguishing it from other objects.
Example (Python):
# Define a class
class Car:
# Constructor method to initialize attributes
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
# Method to get car info
def get_info(self):
return f"{self.year} {self.make} {self.model}"
# Create objects (instances) of the Car class
car1 = Car("Toyota", "Camry", 2020)
car2 = Car("Tesla", "Model S", 2022)
# Access attributes and call methods
print(car1.get_info()) # Output: 2020 Toyota Camry
print(car2.get_info()) # Output: 2022 Tesla Model S
In this example, Car
is a class defining the blueprint for cars. car1
and car2
are objects (instances) of the Car
class, each with its own attributes (make, model, year) and methods (get_info).
2. Encapsulation
Encapsulation is one of the fundamental principles of object-oriented programming (OOP) that involves bundling data and methods that operate on the data into a single unit, called a class. This unit controls access to the data, preventing direct modification from outside the class’s scope and ensuring data integrity.
Example (Python):
class Car:
def __init__(self, make, model):
self.__make = make # private attribute
self.__model = model # private attribute
def get_make(self):
return self.__make
def get_model(self):
return self.__model
def set_make(self, make):
self.__make = make
def set_model(self, model):
self.__model = model
# Creating an instance of Car
my_car = Car("Toyota", "Camry")
# Accessing attributes using methods
print(my_car.get_make()) # Output: Toyota
print(my_car.get_model()) # Output: Camry
# Attempting to access private attributes directly will result in an error
# print(my_car.__make) # This would raise an AttributeError
# Modifying attributes using setter methods
my_car.set_make("Honda")
my_car.set_model("Accord")
# Accessing modified attributes
print(my_car.get_make()) # Output: Honda
print(my_car.get_model()) # Output: Accord
In this example, the Car
class encapsulates the make
and model
attributes, making them private by prefixing them with double underscores (__
). Access to these attributes is provided through getter and setter methods, allowing controlled modification and access to the data. This encapsulation protects the internal state of the Car
class from unintended external modifications, promoting data integrity and making the class easier to maintain and understand.
3. Inheritance
Inheritance in object-oriented programming is a mechanism by which a new class can be derived from an existing class. The new class inherits all the properties and behaviors (methods) of the existing class and can also add its own unique properties and behaviors.
Example (Python):
class Animal:
def __init__(self, species):
self.species = species
def speak(self):
print("I am an animal.")
class Dog(Animal):
def __init__(self, breed):
super().__init__("Dog")
self.breed = breed
def speak(self):
print("Woof!")
class Cat(Animal):
def __init__(self, breed):
super().__init__("Cat")
self.breed = breed
def speak(self):
print("Meow!")
# Creating instances
dog = Dog("Labrador")
cat = Cat("Persian")
# Using inherited methods
dog.speak() # Output: Woof!
cat.speak() # Output: Meow!
In this example, Animal
is the base class with a speak()
method. Dog
and Cat
are subclasses of Animal
and inherit the speak()
method. They also have their own unique attributes (breed
) and override the speak()
method with their specific sounds. This demonstrates how inheritance allows the subclasses to reuse and customize functionality from the base class.
4. Polymorphism
Polymorphism, in object-oriented programming, refers to the ability of different objects to respond to the same message or method invocation in different ways. This means that objects of different classes can be treated as objects of a common superclass, and the appropriate method for the specific object will be called based on its actual class.
Example (Python):
class Animal:
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
# Function that takes an Animal object and makes it speak
def make_speak(animal):
return animal.speak()
# Creating instances
dog = Dog()
cat = Cat()
# Calling the function with different objects
print(make_speak(dog)) # Output: Woof!
print(make_speak(cat)) # Output: Meow!
In this example, both Dog
and Cat
classes inherit from the Animal
class and override its speak()
method. When we call make_speak()
function with different objects, polymorphism allows each object to respond to the speak()
method call differently based on its actual class.
5. Abstraction
Abstraction in Object-Oriented Programming (OOP) is a fundamental concept that allows you to focus on essential details while hiding unnecessary implementation details. It involves creating classes with a clear separation between their external behavior (public interface) and internal implementation (private details). This separation helps in managing complexity, enhancing security, and promoting code reusability.
Example (Python):
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
@abstractmethod
def perimeter(self):
pass
class Rectangle(Shape):
def __init__(self, length, width):
self.length = length
self.width = width
def area(self):
return self.length * self.width
def perimeter(self):
return 2 * (self.length + self.width)
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius * self.radius
def perimeter(self):
return 2 * 3.14 * self.radius
# Using abstraction
rectangle = Rectangle(5, 4)
print("Rectangle area:", rectangle.area())
print("Rectangle perimeter:", rectangle.perimeter())
circle = Circle(3)
print("Circle area:", circle.area())
print("Circle perimeter:", circle.perimeter())
In this example, the Shape
class is an abstract base class defining abstract methods area()
and perimeter()
. The Rectangle
and Circle
classes inherit from Shape
and provide concrete implementations of these methods. Users can create instances of Rectangle
and Circle
and use their area()
and perimeter()
methods without worrying about their internal implementations. This encapsulation of details is an example of abstraction in OOP.
Happy Learning – If you require any further information, feel free to contact me.