Vinay is a student of Engineering college. Since it was time for his college fest he was associated with a task to keep the records of all the events organised. He was finding it difficult to manage the records about the event details manually so he thought of writing a code to keep the record of all the events and other details. Vinay started coding but he is finding bit difficult to get along with it. Help Vinay to write a code according to his requirements (specified in Input format).
Create a class named Event in ‘Event.py’ which has the following attributes,
Attributes | Datatype |
name | str |
detail | str |
type | str |
organiser | str |
attendees_count | int |
projected_expenses | float |
Hint :
Include __init__() constructor
Example:
def __init__(self,name,detail,type,organiser,attendees_count,projected_expenses)
Create main file ‘Main.py’
Obtain input from the user.
Create a new object for the class Event from the input and display the details by __str__() method.
Note: Display single digit after a decimal point.
Input Format:
Input consists of 4 strings indicating Event name, event details, event type and Event organiser name.
Followed by an integer value indicating count of people who attended the event.
And a double value indicating the projected expenses.
Output Format:
The output displays all the details of the event taken as Inputs from user.
Refer Input and Output formats for better understanding.
[All text in bold corresponds to the input and rest corresponds to output]
Sample Input/Output:
Enter the Event Name
Book expo
Enter the Event Details
special discount sale
Enter the Event Type
Reading
Enter the Organiser Name
Ramachandran
Enter the Attendees Count
25
Enter the Projected Expense
5000
Name: Book expo
Detail: special discount sale
Type: Reading
Organiser: Ramachandran
Attendees Count: 25
Projected Expense: 5000.0
Solution:
NameError: name ‘raw_input’ is not defined
change ‘raw_input’ to input
class Event:
def __init__(self, name, detail, type, organiser, attendees_count, projected_expenses):
self.name = name
self.detail = detail
self.type = type
self.organiser = organiser
self.attendees_count = attendees_count
self.projected_expenses = projected_expenses
def __str__(self):
return "Name: {self.name}\nDetail: {self.detail}\nType: {self.type}\nOrganiser: {self.organiser}\nAttendees Count: {self.attendees_count}\nProjected Expense:{self.projected_expenses:.1f}".format(self=self)
from Event import Event
print("Enter the Event Name")
name = raw_input()
print("Enter the Event Details")
detail = raw_input()
print("Enter the Event Type")
type = raw_input()
print("Enter the Organiser Name")
organiser = raw_input()
print("Enter the Attendees Count")
attendees_count = int(input())
print("Enter the Projected Expense")
projected_expenses = float(input())
# pass vakue to class
obj = Event(name, detail, type, organiser, attendees_count, projected_expenses)
print(obj)
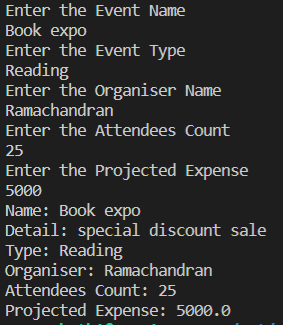
Happy Learning – If you require any further information, feel free to contact me.
i am getting error