Java is a popular programming language that is widely used for building various types of applications, such as web, mobile, and desktop. It is an object-oriented programming language, which means that it is based on the concept of “objects”, which have properties and methods that can be used to perform certain actions.
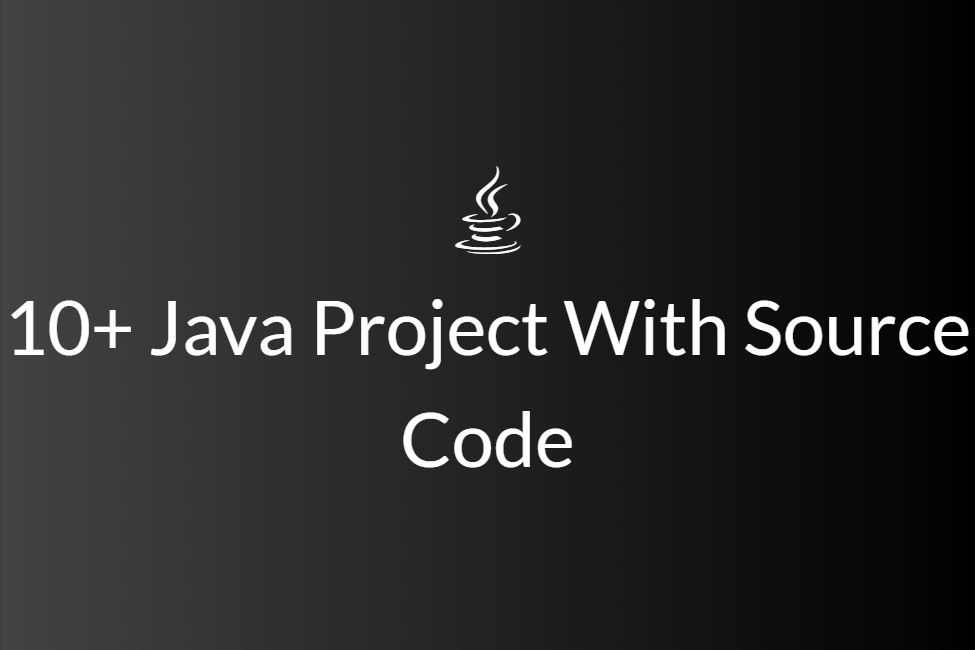
Java was first released in 1995 by Sun Microsystems (now part of Oracle) and has since become one of the most widely used programming languages in the world. It is known for its “Write once, run anywhere” (WORA) principle, meaning that Java code can be written once and run on any platform that supports Java without modification. This is achieved through the use of the Java Virtual Machine (JVM), which interprets the Java bytecode and executes it on the specific platform.
Java is also known for its rich set of libraries and frameworks, which provide pre-built functionality that can be used to quickly build robust and feature-rich applications. Some of the most popular Java libraries and frameworks include Spring, Hibernate, and Apache Struts.
10+ Java Project With Source Code
There are many different types of Java projects that you can create, depending on your interests and skills. Here are a few ideas for Java projects that you might find interesting and challenging:
1. Currency Converter
There are a few different ways to implement a currency converter in Java, but one basic approach would be to use the Java Currency class to handle the currency information, and then use a conversion rate (either hard-coded or obtained from an external source) to convert between currencies. Here is some sample code for a simple currency converter that converts between US dollars and euros:
import java.util.Currency;
import java.util.Locale;
public class CurrencyConverter {
public static void main(String[] args) {
double amount = 100.0;
Currency usd = Currency.getInstance(Locale.US);
Currency eur = Currency.getInstance(Locale.FRANCE);
double conversionRate = 0.8; // 1 USD = 0.8 EUR
double convertedAmount = amount * conversionRate;
System.out.println(amount + " " + usd.getCurrencyCode() + " = " + convertedAmount + " " + eur.getCurrencyCode());
}
}
2.Number Guessing Game
A Java number guessing game would involve the computer randomly generating a number and the player guessing what the number is. The program would then give the player feedback on whether their guess is too high or too low until the player correctly guesses the number. Here is an example of simple number guessing game:
import java.util.Random;
import java.util.Scanner;
public class NumberGuessingGame {
public static void main(String[] args) {
Random rand = new Random();
int numberToGuess = rand.nextInt(100) + 1;
int numberOfGuesses = 0;
Scanner input = new Scanner(System.in);
while (true) {
System.out.print("Guess a number between 1 and 100: ");
int guess = input.nextInt();
numberOfGuesses++;
if (guess == numberToGuess) {
System.out.println("Congratulations! You guessed the number in " + numberOfGuesses + " tries.");
break;
} else if (guess < numberToGuess) {
System.out.println("Too low! Try again.");
} else {
System.out.println("Too high! Try again.");
}
}
input.close();
}
}
3.ATM Interface
An ATM interface in Java is a software interface that allows users to interact with an ATM (Automatic Teller Machine) using the Java programming language. This interface would typically include buttons or menu options for performing common ATM transactions such as withdrawing cash, depositing money, checking account balances, and transferring funds. The interface could be designed to run on a computer or mobile device, and may use a GUI (graphical user interface) or a command-line interface. The implementation of the interface would involve interacting with the ATM’s backend systems to perform the desired transactions.
public interface ATM {
public void withdraw(double amount);
public void deposit(double amount);
public double checkBalance();
public void viewTransactionHistory();
}
public class BankAccount implements ATM {
private double balance;
private List<String> transactionHistory;
public BankAccount(double initialBalance) {
balance = initialBalance;
transactionHistory = new ArrayList<>();
}
public void withdraw(double amount) {
if (amount > balance) {
throw new InsufficientFundsException();
}
balance -= amount;
transactionHistory.add("Withdrew: " + amount);
}
public void deposit(double amount) {
balance += amount;
transactionHistory.add("Deposited: " + amount);
}
public double checkBalance() {
return balance;
}
public void viewTransactionHistory() {
for (String s : transactionHistory) {
System.out.println(s);
}
}
}
4. Data Visualization Software
There are many libraries available in Java for data visualization, such as:
- JFreeChart: a popular open-source library for creating charts and graphs in Java.
- Apache POI: a library for working with Microsoft Office file formats, including Excel.
- iText: a library for creating and manipulating PDF documents.
- Apache PDFBox: a library for working with PDF documents.
- JavaFX: a Java-based framework for creating desktop and web-based applications, including data visualization.
Here’s an example of how you might use JFreeChart to create a bar chart:
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartFrame;
import org.jfree.chart.JFreeChart;
import org.jfree.data.general.DefaultPieDataset;
public class BarChart {
public static void main(String[] args) {
DefaultPieDataset dataset = new DefaultPieDataset();
dataset.setValue("Category 1", 43.2);
dataset.setValue("Category 2", 27.9);
dataset.setValue("Category 3", 79.5);
JFreeChart chart = ChartFactory.createBarChart(
"Comparison between Categories",
"Category",
"Percentage",
dataset);
ChartFrame frame = new ChartFrame("Bar Chart", chart);
frame.pack();
frame.setVisible(true);
}
}
5. Tic-Tac-Toe Game
To create a Tic Tac Toe game in Java, you would need to use a 2D array to represent the game board and use loops and conditional statements to check for winning conditions. You would also need to create methods for players to make their moves and to check the status of the game (e.g. if someone has won or if it’s a tie). Additionally, you would need to create a user interface for the game, such as a simple text-based interface or a graphical interface using a library like JavaFX.
import java.util.Scanner;
public class TicTacToe {
static char[][] board = {{'1', '2', '3'}, {'4', '5', '6'}, {'7', '8', '9'}};
static boolean xTurn = true;
static boolean gameOver = false;
public static void main(String[] args) {
printBoard();
while (!gameOver) {
getInput();
printBoard();
checkWin();
}
}
public static void printBoard() {
for (int i = 0; i < 3; i++) {
System.out.println();
for (int j = 0; j < 3; j++) {
System.out.print(board[i][j] + " ");
}
}
System.out.println();
}
public static void getInput() {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a number: ");
int num = scanner.nextInt();
if (num >= 1 && num <= 9) {
if (xTurn) {
board[(num - 1) / 3][(num - 1) % 3] = 'X';
xTurn = false;
} else {
board[(num - 1) / 3][(num - 1) % 3] = 'O';
xTurn = true;
}
}
}
public static void checkWin() {
// check rows
for (int i = 0; i < 3; i++) {
if (board[i][0] == board[i][1] && board[i][1] == board[i][2]) {
if (board[i][0] == 'X') {
System.out.println("X wins!");
gameOver = true;
} else if (board[i][0] == 'O') {
System.out.println("O wins!");
gameOver = true;
}
}
}
// check columns
for (int i = 0; i < 3; i++) {
if (board[0][i] == board[1][i] && board[1][i] == board[2][i]) {
if (board[0][i] == 'X') {
System.out.println("X wins!");
gameOver = true;
} else if (board[0][i] == 'O') {
System.out.println("O wins!");
gameOver = true;
}
}
}
// check diagonals
if (board[0][0] == board[1][1] && board[1][1] == board[2][2]) {
if (board[0][0] == 'X') {
System.out.println("X wins!");
gameOver = true;
} else if (board[0][0] == 'O') {
System.out.println("O wins!");
gameOver = true;
}
}
6. Bank Management Software
import java.util.Scanner;
public class BankApplication {
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
System.out.println("Enter your 'Name' and 'CustomerId' to access your Bank account:");
String name=sc.nextLine();
String customerId=sc.nextLine();
BankAccount obj1=new BankAccount(name,customerId);
obj1.menu();
}
}
class BankAccount{
double bal;
double prevTrans;
String customerName;
String customerId;
BankAccount(String customerName,String customerId){
this.customerName=customerName;
this.customerId=customerId;
}
void deposit(double amount){
if(amount!=0){
bal+=amount;
prevTrans=amount;
}
}
void withdraw(double amt){
if(amt!=0 && bal>=amt){
bal-=amt;
prevTrans=-amt;
}
else if(bal<amt){
System.out.println("Bank balance insufficient");
}
}
void getPreviousTrans(){
if(prevTrans>0){
System.out.println("Deposited: "+prevTrans);
}
else if(prevTrans<0){
System.out.println("Withdrawn: "+Math.abs(prevTrans));
}
else{
System.out.println("No transaction occured");
}
}
void menu(){
char option;
Scanner sc=new Scanner(System.in);
System.out.println("Welcome "+customerName);
System.out.println("Your ID:"+customerId);
System.out.println("\n");
System.out.println("a) Check Balance");
System.out.println("b) Deposit Amount");
System.out.println("c) Withdraw Amount");
System.out.println("d) Previous Transaction");
System.out.println("e) Exit");
do{
System.out.println("********************************************");
System.out.println("Choose an option");
option=sc.next().charAt(0);
System.out.println("\n");
switch (option){
case 'a':
System.out.println("......................");
System.out.println("Balance ="+bal);
System.out.println("......................");
System.out.println("\n");
break;
case 'b':
System.out.println("......................");
System.out.println("Enter a amount to deposit :");
System.out.println("......................");
double amt=sc.nextDouble();
deposit(amt);
System.out.println("\n");
break;
case 'c':
System.out.println("......................");
System.out.println("Enter a amount to Withdraw :");
System.out.println("......................");
double amtW=sc.nextDouble();
withdraw(amtW);
System.out.println("\n");
break;
case 'd':
System.out.println("......................");
System.out.println("Previous Transaction:");
getPreviousTrans();
System.out.println("......................");
System.out.println("\n");
break;
case 'e':
System.out.println("......................");
break;
default:
System.out.println("Choose a correct option to proceed");
break;
}
}while(option!='e');
System.out.println("Thank you for using our banking services");
}
}
7. Temperature Converter
public class Temperature_converter extends javax.swing.JFrame {
//Variable Declaration
private javax.swing.JButton jButton1;
private javax.swing.JButton jButton2;
private javax.swing.JButton jButton3;
private javax.swing.JCheckBox jCheckBox2;
private javax.swing.JComboBox<String> jComboBox1;
private javax.swing.JComboBox<String> jComboBox2;
private javax.swing.JLabel jLabel1;
private javax.swing.JPanel jPanel2;
private javax.swing.JSpinner jSpinner1;
private javax.swing.JTextField jTextField1;
private javax.swing.JTextField jTextField2;
public Temperature_converter() {
initComponents();
}
private void initComponents() {
jCheckBox2 = new javax.swing.JCheckBox();
jSpinner1 = new javax.swing.JSpinner();
jPanel2 = new javax.swing.JPanel();
jLabel1 = new javax.swing.JLabel();
jComboBox1 = new javax.swing.JComboBox<>();
jComboBox2 = new javax.swing.JComboBox<>();
jTextField1 = new javax.swing.JTextField();
jTextField2 = new javax.swing.JTextField();
jButton1 = new javax.swing.JButton();
jButton2 = new javax.swing.JButton();
jButton3 = new javax.swing.JButton();
jCheckBox2.setText("jCheckBox2");
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
setTitle("Tempertaure Converter");
jPanel2.setBackground(new java.awt.Color(51, 51, 51));
jLabel1.setBackground(new java.awt.Color(255, 255, 255));
jLabel1.setFont(new java.awt.Font("Segoe UI", 1, 24)); // NOI18N
jLabel1.setForeground(new java.awt.Color(255, 255, 255));
jLabel1.setText("Temperature Converter ");
javax.swing.GroupLayout jPanel2Layout = new javax.swing.GroupLayout(jPanel2);
jPanel2.setLayout(jPanel2Layout);
jPanel2Layout.setHorizontalGroup(
jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel2Layout.createSequentialGroup()
.addGap(142, 142, 142)
.addComponent(jLabel1)
.addContainerGap(161, Short.MAX_VALUE))
);
jPanel2Layout.setVerticalGroup(
jPanel2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel2Layout.createSequentialGroup()
.addGap(19, 19, 19)
.addComponent(jLabel1)
.addContainerGap(23, Short.MAX_VALUE))
);
jComboBox1.setModel(new javax.swing.DefaultComboBoxModel<>(new String[] { "Celsius", "Fahrenheit" }));
jComboBox2.setModel(new javax.swing.DefaultComboBoxModel<>(new String[] { "Celsius", "Fahrenheit" }));
jTextField2.setEditable(false);
jButton1.setFont(new java.awt.Font("Segoe UI", 3, 14)); // NOI18N
jButton1.setText("Convert");
jButton1.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton1ActionPerformed(evt);
}
});
jButton2.setFont(new java.awt.Font("Segoe UI", 3, 14)); // NOI18N
jButton2.setText("Clear");
jButton2.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton2ActionPerformed(evt);
}
});
jButton3.setFont(new java.awt.Font("Segoe UI", 3, 14)); // NOI18N
jButton3.setText("Exit");
jButton3.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton3ActionPerformed(evt);
}
});
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(50, 50, 50)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jComboBox2, 0, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jComboBox1, 0, 116, Short.MAX_VALUE))
.addGap(133, 133, 133)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jTextField1, javax.swing.GroupLayout.PREFERRED_SIZE, 97, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jTextField2, javax.swing.GroupLayout.PREFERRED_SIZE, 97, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jButton1)
.addGap(30, 30, 30)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jButton3)
.addComponent(jButton2))
.addGap(29, 29, 29))
.addComponent(jPanel2, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(jPanel2, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(46, 46, 46)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jComboBox1, javax.swing.GroupLayout.PREFERRED_SIZE, 34, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jTextField1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(3, 3, 3)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jButton1)
.addComponent(jButton2))
.addGap(18, 18, 18)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jComboBox2, javax.swing.GroupLayout.PREFERRED_SIZE, 33, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jTextField2, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jButton3)
.addGap(37, 37, 37))
);
pack();
}//
private void jButton3ActionPerformed(java.awt.event.ActionEvent evt) {
System.exit(0);
}
private void jButton2ActionPerformed(java.awt.event.ActionEvent evt) {
jTextField1.setText("");
jTextField2.setText("");
}
private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) {
String val1=(String)jComboBox1.getSelectedItem();
String val2=(String)jComboBox2.getSelectedItem();
if(val1.equals("Celsius") && val2.equals("Fahrenheit")){
double cel = Double.parseDouble(jTextField1.getText());
double fah = (double)((9.0/5.0)*cel + 32);
jTextField2.setText(String.valueOf(fah));
}
else if(val1.equals("Celsius") && val2.equals("Celsius"))
{
double c = Double.parseDouble(jTextField1.getText());
jTextField2.setText(String.valueOf(c));
}
if(val1.equals("Fahrenheit") && val2.equals("Celsius"))
{
double f = Double.parseDouble(jTextField1.getText());
double c = (double)((f - 32)*(5.0/9.0));
jTextField2.setText(String.valueOf(Math.round(c)));
}
else if(val1.equals("Fahrenheit") && val2.equals("Fahrenheit"))
{
double f = Double.parseDouble(jTextField1.getText());
jTextField2.setText(String.valueOf(Math.round(f)));
}
}
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(Temperature_converter.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(Temperature_converter.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(Temperature_converter.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(Temperature_converter.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new Temperature_converter().setVisible(true);
}
});
}
}
8. Digital Clock
Another simple and easy-to-implement project that you can show off is a digital clock. Some knowledge of UI design is needed here if a visually pleasing design is wanted (which does help a lot!). This requires some event handling as well as periodic execution functions to achieve the desired effect.
Extra features such as other modes, including that of a stopwatch and a counter can be made to improve the project’s output. If that itself isn’t challenging enough, adding the option to move between time zones and show the time according to the time zone selected is certainly going to be a challenge.
import javax.swing.*;
import java.awt.*;
import java.text.SimpleDateFormat;
import java.util.Calendar;
public class Clock extends JFrame {
Calendar calendar;
SimpleDateFormat timeFormat;
SimpleDateFormat dayFormat;
SimpleDateFormat dateFormat;
JLabel timeLabel;
JLabel dayLabel;
JLabel dateLabel;
String time;
String day;
String date;
Clock() {
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setTitle("Digital Clock");
this.setLayout(new FlowLayout());
this.setSize(350, 220);
this.setResizable(false);
timeFormat = new SimpleDateFormat("hh:mm:ss a");
dayFormat=new SimpleDateFormat("EEEE");
dateFormat=new SimpleDateFormat("dd MMMMM, yyyy");
timeLabel = new JLabel();
timeLabel.setFont(new Font("SANS_SERIF", Font.PLAIN, 59));
timeLabel.setBackground(Color.BLACK);
timeLabel.setForeground(Color.WHITE);
timeLabel.setOpaque(true);
dayLabel=new JLabel();
dayLabel.setFont(new Font("Ink Free",Font.BOLD,34));
dateLabel=new JLabel();
dateLabel.setFont(new Font("Ink Free",Font.BOLD,30));
this.add(timeLabel);
this.add(dayLabel);
this.add(dateLabel);
this.setVisible(true);
setTimer();
}
public void setTimer() {
while (true) {
time = timeFormat.format(Calendar.getInstance().getTime());
timeLabel.setText(time);
day = dayFormat.format(Calendar.getInstance().getTime());
dayLabel.setText(day);
date = dateFormat.format(Calendar.getInstance().getTime());
dateLabel.setText(date);
try {
Thread.sleep(1000);
} catch (Exception e) {
e.getStackTrace();
}
}
}
public static void main(String[] args) {
new Clock();
}
}
9.Snake Game using Java
In our childhood, nearly all of us have enjoyed playing classic snake games. Now we will try to enhance with the help of Java concepts. The concept appears to be easy but it is not that effortless to implement.
One ought to comprehend the OOPs concept in detail to execute this effectively. Furthermore, ideas of Java Swing are used to create this application. The application should comprise the following functionalities:
- The Snake will have the ability to move in all four directions.
- The snake’s length grows as it eats food.
- When the snake crosses itself or strikes the perimeter of the box, the game is marked over.
- Food is always given at different positions.
public class Game {
public static final int DIRECTION_NONE = 0, DIRECTION_RIGHT = 1,
DIRECTION_LEFT = -1, DIRECTION_UP = 2, DIRECTION_DOWN = -2;
private Snake snake;
private Board board;
private int direction;
private boolean gameOver;
public Game(Snake snake, Board board)
{
this.snake = snake;
this.board = board;
}
public Snake getSnake()
{
return snake;
}
public void setSnake(Snake snake)
{
this.snake = snake;
}
public Board getBoard()
{
return board;
}
public void setBoard(Board board)
{
this.board = board;
}
public boolean isGameOver()
{
return gameOver;
}
public void setGameOver(boolean gameOver)
{
this.gameOver = gameOver;
}
public int getDirection()
{
return direction;
}
public void setDirection(int direction)
{
this.direction = direction;
}
// We need to update the game at regular intervals,
// and accept user input from the Keyboard.
public void update()
{
System.out.println("Going to update the game");
if (!gameOver) {
if (direction != DIRECTION_NONE) {
Cell nextCell = getNextCell(snake.getHead());
if (snake.checkCrash(nextCell)) {
setDirection(DIRECTION_NONE);
gameOver = true;
}
else {
snake.move(nextCell);
if (nextCell.getCellType() == CellType.FOOD) {
snake.grow();
board.generateFood();
}
}
}
}
}
private Cell getNextCell(Cell currentPosition)
{
System.out.println("Going to find next cell");
int row = currentPosition.getRow();
int col = currentPosition.getCol();
if (direction == DIRECTION_RIGHT) {
col++;
}
else if (direction == DIRECTION_LEFT) {
col--;
}
else if (direction == DIRECTION_UP) {
row--;
}
else if (direction == DIRECTION_DOWN) {
row++;
}
Cell nextCell = board.getCells()[row][col];
return nextCell;
}
public static void main(String[] args)
{
System.out.println("Going to start game");
Cell initPos = new Cell(0, 0);
Snake initSnake = new Snake(initPos);
Board board = new Board(10, 10);
Game newGame = new Game(initSnake, board);
newGame.gameOver = false;
newGame.direction = DIRECTION_RIGHT;
// We need to update the game at regular intervals,
// and accept user input from the Keyboard.
// here I have just called the different methods
// to show the functionality
for (int i = 0; i < 5; i++) {
if (i == 2)
newGame.board.generateFood();
newGame.update();
if (i == 3)
newGame.direction = DIRECTION_RIGHT;
if (newGame.gameOver == true)
break;
}
}
}
10. Chat Bot Using Java
import java.util.Scanner;
public class Chatbot {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Hello, I'm a chatbot. What's your name?");
String name = scanner.nextLine();
System.out.println("Nice to meet you, " + name + ". How can I help you today?");
String question = scanner.nextLine();
System.out.println("I'm sorry, I'm not able to understand your question.");
}
}